Javascript : Variables and Datatypes

Rawan Amr Abdelsattar
Posted on October 16, 2021
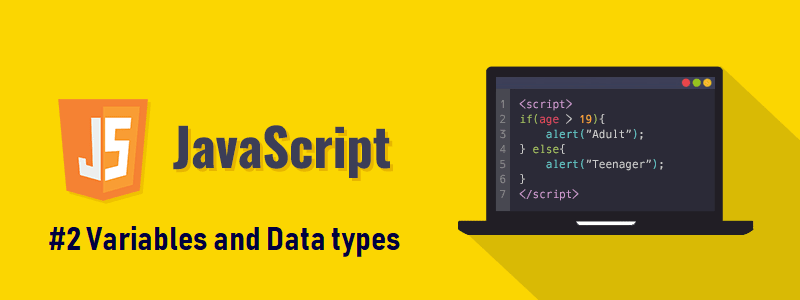
What is a Variable?!
A variable is a container with a label (name) where you store a certain value ( a piece of data ) to use it in your code.Declaring variables and assigning values to them
To declare a variable is to give it a name, you have to use one of these keywords:
- var : most common key to declare variables.
- let : only visible within the block where it's declared.
- const : used for constant values and cannot be changed , it will cause an error if you tried to do so.
var myVariable ;
let myName ;
const pi ;
Variable naming conventions
To name a variable in javascript , you should follow some rules:- javascript is case sensitive which means that the letters' case matters (VARIABLE isn't the same as Variable and also not as variable).
- you have to use camelCase (example: myName, schoolYear, etc.)
- You can add numbers but not at the beginning (whatever , who will name a variable 2myVarName, this won't satisfy the rule n.o. 2)
- you cannot use hyphens " - " or spaces but you can use underscores " _ " instead (note : underscores can be used as the first character in a variable name).
- You cannot use any of the reserved keywords (like : var, let, const, if, while, for and so on) . don't worry if you don't know a lot of keywords , if you tried to use one it will be highlighted as a keyword and results in an error.
Assigning values to variables
To assign a value to a variable, use the assignment operator " = " (equal sign).// Declaring variables
var myVariable ;
let myName ;
const pi ;
// assigning values to variables
myVariable = 25;
myName= "Rawan";
pi = 3.14;
Note : you can declare variables and assign values to them on the same line.
// Declaring a variable and assigning a value to it on one line
var myAge = 15;
Datatypes
In Javascript there are a lot of data types, we will discuss the most important and basic ones.Main Datatypes :
- Numbers : they can be integer numbers or floats( numbers with decimal points).
- Strings : any series of characters included between quotes (double quotes " " or single quotes ' ' ).
- Boolean value : has one of the two values true or false .
- null : means nothing.
- undefined : something that hasn't been defined.
- Arrays : can store more than one piece of data.
- Objects : used to store key-value pairs( each property associated with its own value).
Getting output in Javascript
You can output values to the console using console.log() , inside the parenthesis put a variable name or any piece of data to be shown in the console.console.log("Hello World !"); // output : Hello World !
var myScore = 320 ;
console.log(myScore); // output : 320
Notes , Again...
1 . Everything greyed out (not highlighted) after " // " is called a comment , you write them to explain to yourself and others what your code does , you write them using :- // : for inline comments
- /* */ : for multi-line comments
// I am an inline comment
/* I am a
multi-line
comment */
2 . In Javascript we put a semicolon "; " after the end of each line, you don't have to do it all the time, but it's a good practice to do so.
3 . Variables that are declared but not assigned to store any values are called " Uninitialized variables " and have a default value of undefined .
That was all for this tutorial , if you like it leave a comment , like the tutorial and follow me to receive notifications when I post new tutorials
💖 💪 🙅 🚩

Rawan Amr Abdelsattar
Posted on October 16, 2021
Join Our Newsletter. No Spam, Only the good stuff.
Sign up to receive the latest update from our blog.
Related
javascript Exploring Node.js: What Makes It a Powerful Choice for Server-Side Development
November 6, 2024